If you've been wanting to build a smart WhatsApp bot but didn’t want to get tangled in SDKs, cloud functions, or bloated frameworks—this is for you.
You don’t need a massive codebase or a weekend to build a smart WhatsApp bot. All you need is Python, GPT‑4.1, Heltar WhatsApp API (available for free), and about 15 minutes.
This guide will walk you through building a fully functional chatbot that:
- Gets messages from WhatsApp via Heltar
- Uses GPT-4.1 to decide what button to show next
- Sends a reply straight to the user
It’s all done with ~30 lines of Python. No filler, no fluff, and no spinning up a giant backend stack. If you’ve built Flask APIs before, this’ll feel familiar. If not, don’t worry—this walkthrough is straight to the point.
Let’s get started.
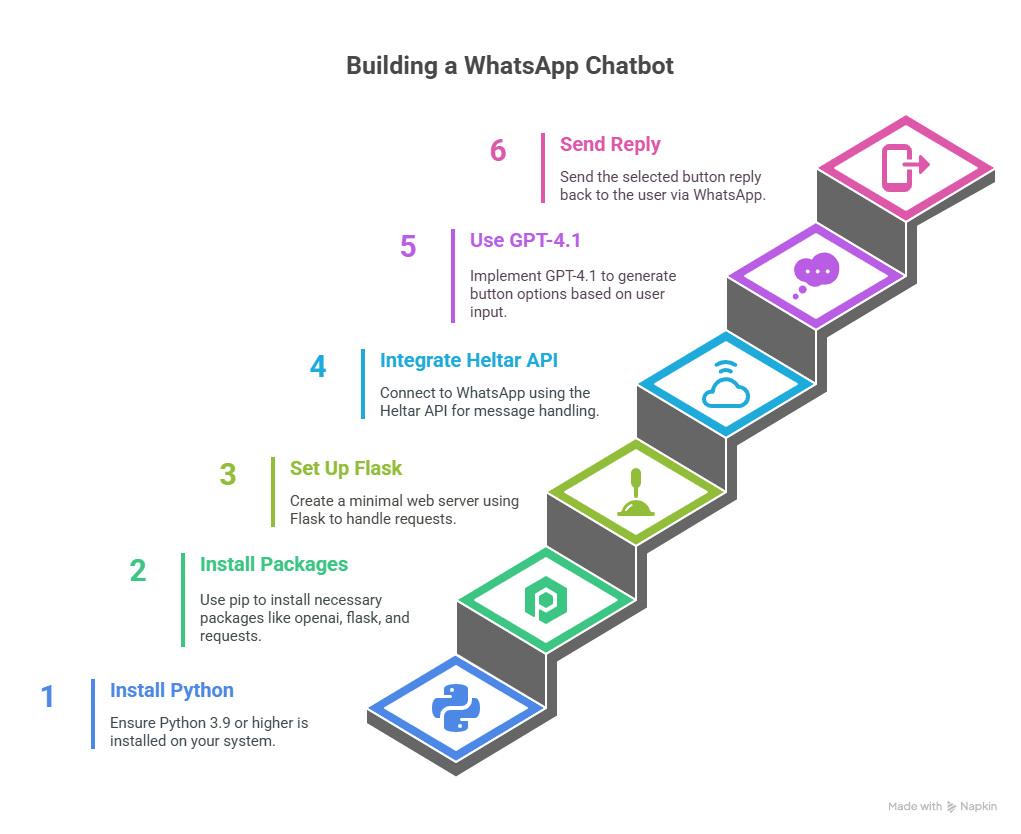
What You’ll Need?
Here’s what we’re working with:
Tool | Why you need it |
Python ≥ 3.9 | Scripting and async-friendly |
openai 1.x | Access to GPT‑4.1 |
Flask | Minimal web server |
Heltar API | Fastest way to get WhatsApp working |
Ngrok (optional) | Test webhooks from localhost |
If you don't have an account on Heltar you can set up an account for free by following this tutorial:
Install the required packages:
pip install openai flask requests python-dotenv
1. Define What Buttons Look Like
We want GPT to respond in a format that lets us generate real buttons in WhatsApp. Here’s a basic JSON schema that tells GPT exactly what kind of response we expect:
quick_reply = {
"name": "quick_reply",
"description": "User must pick one of the provided buttons.",
"parameters": {
"type": "object",
"properties": {
"choice": {
"type": "string",
"enum": ["Approve", "Reject"]
}
},
"required": ["choice"]
}
}
This structure forces GPT to pick between “Approve” or “Reject” when it uses this function. Predictable output is the goal here.
2. The ~30-Line Flask App
Here’s the full working Flask app that connects Heltar, GPT‑4.1, and WhatsApp messages:
from flask import Flask, request
from openai import OpenAI
import os, json, requests, dotenv
dotenv.load_dotenv()
openai = OpenAI()
HELTAR_TOKEN = os.getenv("HELTAR_TOKEN")
HELTAR_URL = "https://api.heltar.io/v1/messages"
app = Flask(__name__)
HISTORY = [] # Replace with DB or Redis in production
@app.route("/whatsapp", methods=["POST"])
def whatsapp_webhook():
inbound = request.json
user_msg = inbound["text"]
chat_id = inbound["chat_id"]
HISTORY.append({"role": "user", "content": user_msg})
chat = openai.chat.completions.create(
model="gpt-4.1-chat-bison",
messages=HISTORY,
functions=[quick_reply],
temperature=0.3
)
reply = chat.choices[0].message
if reply.function_call:
labels = quick_reply["parameters"]["properties"]["choice"]["enum"]
text = "Pick one:"
buttons = [{"type": "reply", "label": l, "payload": l} for l in labels]
payload = {"chat_id": chat_id, "text": text, "buttons": buttons}
else:
text = reply.content
payload = {"chat_id": chat_id, "text": text}
HISTORY.append({"role": reply.role, **reply.dict(exclude_none=True)})
requests.post(HELTAR_URL, json=payload, headers={"Authorization": f"Bearer {HELTAR_TOKEN}"})
return "ok"
3. Run and Connect
Start the Flask app:
python app.py
If you’re testing locally, use ngrok to expose your server:
ngrok http 5000
Then go into your Heltar dashboard and set your webhook to:
https://<your-ngrok-id>.ngrok.io/whatsapp
Now send a WhatsApp message to your Heltar test number. You’ll get a response from GPT‑4.1—and buttons you can actually tap.
Why This Works (and Doesn’t Break)
The magic here is in structured output. Instead of parsing unpredictable text, you specify a clear format GPT must follow. This gives you:
Predictable behavior
Zero need for regex or brittle parsing
Easy chaining of steps (like collecting info, opening support tickets, etc.)
It also means you can swap in new flows without changing your backend much. Just tweak the schema, and you’re good to go.
What to Try Next?
Now that you have the basic bot up and running, here’s a few quick ways to build on it:
Add more button choices for menus
Collect structured user input like email, phone, etc.
Return media URLs—WhatsApp will render images automatically
Need Help?
Ping us anytime—our engineers at Heltar love debugging and will get you unblocked quickly.
You're Done!
You now have a working GPT‑4.1-driven WhatsApp chatbot, complete with tappable buttons, in around 30 lines of Python. Fork it. Extend it. Hook it into your CRM.
For more smart WhatsApp Solutions, check out heltar.com/blogs.